I wanted to learn a new programming language and looked at some of the most popular and well-liked ones, using the 2023 Stack Overflow Developer Survey as a starting point.
I started with studying Rust. Rust is really well liked on Stack Overflow, and I enjoyed learning it. There are a lot of things I like about it, but it focuses so much on efficiency and safety that the code tends to be pretty verbose. It has a lot of features—maybe too many for me. But if I were working on code that needed to be really fast and bullet-proof, I’d learn more Rust.
More recently I’ve been learning Go (aka Golang) using the Tour of Go web tutorial. The developers of Go wanted to merge the best parts of C (fast) and Python (easy), and I feel like they did a pretty good job. I found it much easier to learn than Rust, and I liked how simple they were able to keep the language.
As I came to the conclusion of the tutorial, I used Go to generate a bunch of math-art pictures. Below are the two that I liked the most, with source code (which you can run and edit online at this Tour of Go page).
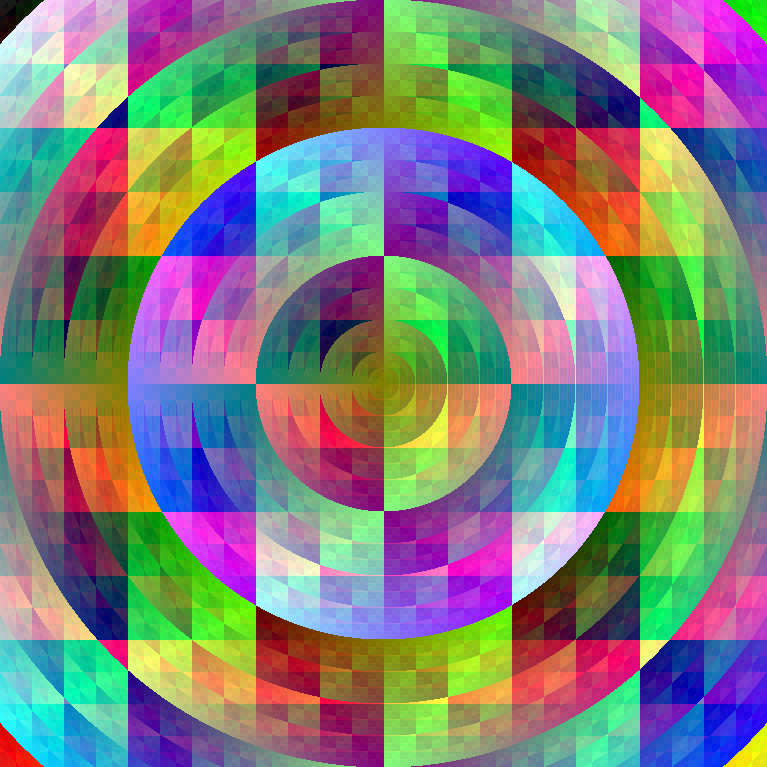
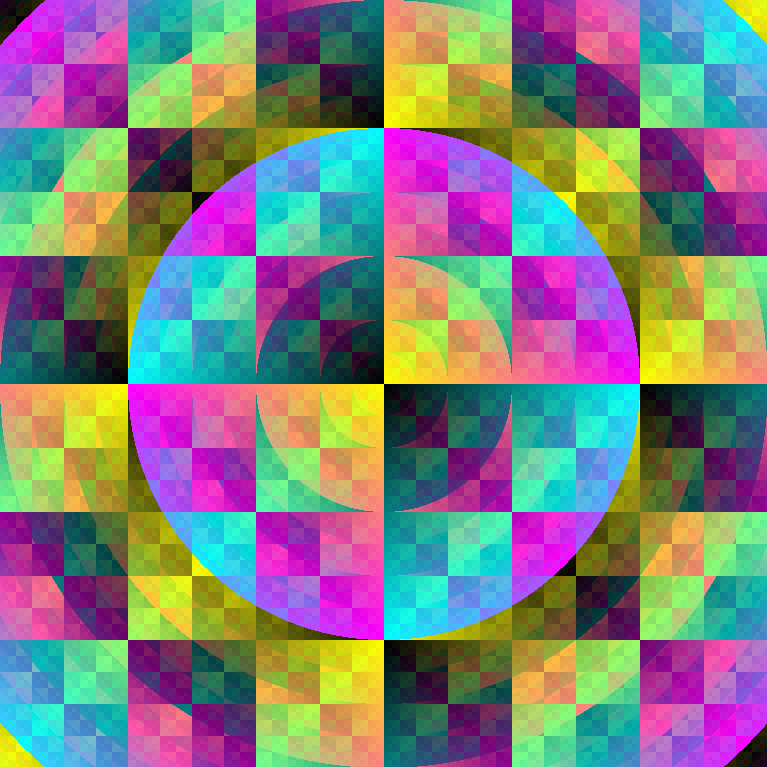
package main import ( "image" "image/color" "math" "golang.org/x/tour/pic" ) type Image struct{} func (i Image) Bounds() image.Rectangle { return image.Rect(0, 0, 767, 767) } func (i Image) ColorModel() color.Model { return color.RGBAModel } // Here's the interesting part: A function that returns the RGBA color at // each (x, y) coordinate. func (i Image) At(x, y int) color.Color { xc, yc := (767.0/2)-float64(x), (767.0/2)-float64(y) d := int(math.Sqrt(xc*xc + yc*yc)) v := (x ^ y) ^ d // Image 1 // return color.RGBA{byte(v), byte(x^y), byte(d), 255} // Image 2 return color.RGBA{byte(v^x), byte(v^y), byte(d), 255} } func main() { m := Image{} pic.ShowImage(m) }